10/17/2013 to 11/06/2014Oct 28
You have a credit card debt of $500. The interest rate on your card is 18.50%. If you were to pay the minimum payment fee of $10.00 per month you want to know how many months it will take and how much you will pay in total. Your output should show your credit card balance by month. i.e.:
- Month 1: $500
- Month 2: $500 + 500*0.1850/12 - 10
You main program should loop until the balance is less than or equal to 0 and then call a function to calculate the balance for the month. You will want to set default values for the Interest Rate, credit card debt and payment amount but allow the user to input different values.
10/17/2013 to 11/06/2014Functions
Oct 21
You can create your own functions.
public void myFuntion(){
//Code goes here
}
public - access modifier
void - return type - use void if nothing returned. Otherwise the data type of what you want to return (i.e. int)
myFunction - the name that you will use to call your function
() - parameters - since this was empty there were no parameters. If you had variable type and name then that is the parameter you are passing. i.e.
public void calcArea(int length, int width){}
{} - this is where you will put the code. If it returns a value you MUST use return somevalue in the function.
10/17/2013 to 11/06/2014U3D1 Arrays
An array holds a series of data.
Declare an array
int[] marks;
Instantiate an array
marks = new int[4]
Initialize the values in an array
marks[0] = 95;
marks[1] = 90;
marks[2] = 98;
marks[3] = 75;
-or-
marks = {95,90,98,75};
Please note - you can use a for loop to quickly loop through each element.
.Length property
marks.Length returns a value of 4 - there are 4 elements. Note that the elements are numbered starting at 0 so to access the last element you use the index value of 3.
for(int i = 0; i < marks.Length; i++)
{
rtbOutput.Text += marks[i].ToString() + "\n";
}
10/2/2013 to 10/15/2013U2D7 Databases
Databases are integral in todays world. SQL (Structured Query Language) is the language of data. Microsoft Access is an good way to learn how releational databases are set up. When you get dealing with a website you will likely have access to a MySql database, SQLite for mobile phones.
Microsoft Access training
9/30/2013 to 10/15/2013U2D5 Constructors
A Constructor is a method that has the same name as the class. Any code that is to run when the instance of the object is created goes here. For example out deck of cards class constructor will initialize the array of cards. View the deck class here.
Object Oriented programming consists of three features:
- Polymorphism
- Encapsulation
- Abstraction
Learn more about OOP and C# here.
9/24/2013 to 10/15/2013U2D1 Save and Open Files
A programmer’s wife sends him to the grocery store with the instructions, “get a loaf of bread, and if they have eggs, get a dozen.” He comes home with a dozen loafs of bread and tells her, “they had eggs.”
Big Idea: Be able to save and open files in programs.
Minds on: How data is stored
Activity: Create Contact book saver
If time we will add the ability to save and open using other formats (xml, serialization)
Consolidation: Show off
9/25/2013 to 10/15/2013
U2D2
We need to finish up reading and writing to files today. Below are two videos that go over file reading and writing operations. Take the time to review them - they are very good!
Here is a UML diagram based on the textbook. Our goal is to create two enum data types. One to represent the suit of a card, the other to represent the face value of a card. For example: 2 of clubs (2 is the face value, clubs is the suit). By the end of the period can you have a UML diagram done and a Windows Form Application that displays a deck of cards by looping and using the enum value?
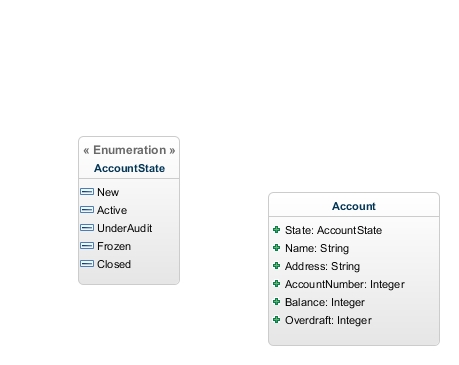
Textbook
For a textbook we will be using a series of powerpoints. You can access the first powerpoints from your TLDSB Google account (I will show you how on the first day of classes if you haven't accessed it before). We will be using Visual Studio Professional (available through Dreamspark).
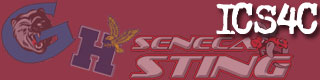
This course further develops students’ computer programming skills. Students will learn object-oriented programming concepts, create object-oriented software solutions, and design graphical user interfaces. Student teams will plan and carry out a software development project using industry-standard programming tools and proper project management techniques. Students will also investigate ethical issues in computing and expand their understanding of environmental issues, emerging technologies, and computer-related careers.
Fun Fridays
App Inventor
Canadian Computing Competition
XNA
- Check your Google Drive account for resources