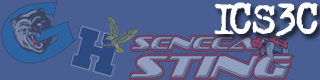
Images
I've had a question about how to add a picture to the screen. If you right click on your project you can add an existing item (the picture in question which is called photo.png). Then for your form you can select the paint event. Here is the code I added to the paint event to draw the image 10 times.
Bitmap b = new Bitmap("photo.png"); for (int i = 0; i < 10; i++) { e.Graphics.DrawImage(b, i*25, 0); }
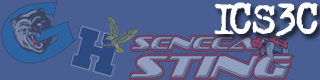
Where did everything go?
To keep things tidy, I've put everything from unit 1 and 2 on the Unit 1 and Unit 2 page.
Planning Tools
Oct 22
Flowcharts
Draw.io is an online drawing tool that makes it easy to create flowcharts. Flowcharts allow you to plan your program by drawing the stages it will follow. Here are the parts of a diagram:
Start
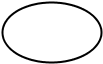
Each function must have a start point. An arrow will point to the next step.
End
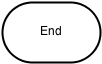
Each function must have an end point.
Input/Output
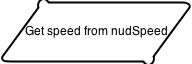
Input (i.e. get value from numericupdown) or Output (i.e. set the text of a label) use this shape.
Calculation

Any calculations or setting of values use the rectangle shape.
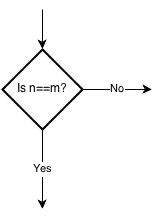
Decisions are used for if statements or loops. Really important that you remember that you can only have two arrows leaving the diamond - everything in a computer is binary - yes/no, true/false. If you use a diamond for a loop, then one of your arrows must point before.
Functions
Oct 21
You can create your own functions.
public void myFuntion(){ //Code goes here }
public - access modifier
void - return type - use void if nothing returned. Otherwise the data type of what you want to return (i.e. int)
myFunction - the name that you will use to call your function
() - parameters - since this was empty there were no parameters. If you had variable type and name then that is the parameter you are passing. i.e.
public void calcArea(int length, int width){}
{} - this is where you will put the code. If it returns a value you MUST use return somevalue in the function.
U3D2 Logic Errors
Oct 18
A logic error (sometimes called a semantic error) is when you have a program that will run but the result is not what you expect.
Examples:
- Using the wrong data type (int when you want a decimal result)
- Using the wrong variable
- Using the wrong operator (< instead of >)
One of the best ways to detect logic errors are manual traces. Turn on line numbering Tools - Options - Text Editor - All Languages and check the line numbering box.
Use a spreadsheet of a piece of paper and keep track of each variable in a column - each line of code would be a row and keep track of the values. You can get the computer to do the work by using Console.WriteLine();
Your challenge
In class you need to go through the two programs Mr. McTavish prepared. Identify the logic errors, fix them and show Mr. McTavish.
Then you will create your own program with an error and have a partner work on identifying the error and the type of error.
U3D2 Run-time Errors
Oct 17
Run-time errorsĀ produce error messages or crashes while the program is running. They occur when correctly written code is asked to perform an impossible task (like trying to put an elephant in a cup).
Examples:
- trying to access the tenth character of a String variable that has only four letters;
- trying to perform a mathematical operation on a variable that is not a number.
- trying to perform a mathematical operation on a variable that is not a number.
- Divide by 0
Strategies:
- Use if statements to ensure data is valid.
- int.TryParse(stringvalue,out intvariable); will convert a string to an int (double has a TryParse method as well). It will return true if it works and false if it doesn't so you can use it in an if statement!
Recap of basic skills
Declare a variable
Variables need to be declared before they are used. You need to set the type (i.e. words - string, number with decimal - double, number with no decimal - int, true/false - bool) and then give the variable a name (letters only, no spaces)
string firstName; int age; double amountPaid;
Assign a value to a variable
You can't use a variable until you have assigned it a value. You need to use the variablename then = then the value (which can be an expression). Examples:
firstName = "Bob"; age = 16; amountPaid = 100*1.13;
Get Input from the user
To get a string you need a text box, to get a number use a numeric up down control. Examples:
firstName = txtName.Text; age = (int)nudAge.Value; amountPaid = (double)nudPaid.Value;
Output
Labels, text boxes and Rich Text Boxes all have the .Text property that you can assign a string to. Other types of variables can be converted to strings using .ToString() method. Example:
txtOutput.Text = firstName + " is " + age.ToString() + " years old.";
U3D12 Oct 16
Syntax Error
Syntax errors are errors that will prevent your program from compiling. Visual Studio points out the errors.
Common errors include:
- Improper use of semicolons
- Having too many or too few brackets or braces
- Spelling mistakes (capitalization matters)
- Using a comma instead of a semicolon
You will get a build error - if you're luckly it will tell you what you need. Sometimes the error is actually caused before.